Every software project faces the inevitable challenge of dependency management. Over time, dependencies become outdated, leading to security vulnerabilities. Others go unused, needlessly bloating build times. For many development teams, addressing these issues means running manual scripts, reviewing output files, and hoping nothing falls through the cracks.
I recently transformed this error-prone manual process into an automated solution using a few tools on GitHub—GitHub Copilot, GitHub Actions, and Dependabot, to be specific (just in case you’re wondering). Here’s how you can do the same!
So, let’s jump in (and make sure to watch the video above, too!).
The problem with manual dependency audits
Most teams start with a simple approach to dependency management. This often includes a Bash script that runs periodically. Here’s what our manual script looks like:
#!/bin/bash
echo "Starting manual dependency audit..."
echo "Installed dependencies:"
npm list --depth=0 > deps.txt
cat deps.txt
echo -e "\nChecking outdated dependencies..."
npm outdated > outdated.txt
cat outdated.txt
echo -e "\nLooking for potentially unused dependencies..."
for dep in $(npm list --depth=0 | grep '├──' | cut -d' ' -f2 | cut -d@ -f1); do
if ! find . -type f -name "*.js" -o -name "*.tsx" -o -name "*.ts" | xargs grep -l "$dep" > /dev/null 2>&1; then
echo "$dep might be unused"
fi
done
echo "Done! Check deps.txt and outdated.txt manually. Phew that was a lot of work!"
This approach has several limitations, including:
- It’s manual, so someone has to remember to run it (and let’s be honest, I often forget to run these in my own codebases).
- The unused dependency check is crude, and often inaccurate.
- Results are scattered across multiple output files.
- It’s not integrated with workflows or CI/CD pipelines.
There has to be a better way than this—right?
How to simplify dependency audits on GitHub
Luckily there is, in fact, a better solution than manual Bash script if you’re working on GitHub—and it starts with using a combination of our AI developer tool, GitHub Copilot, our automation and CI/CD tool. GitHub Actions, and Dependabot, our automated dependency tool.
Here’s a step-by-step guide you can use to do this.
Step 1: Use GitHub Copilot to create the action
Agent mode takes GitHub Copilot from suggesting code to owning tasks, like transforming our bash script into a GitHub Actions workflow.
Here is our prompt:
“Create a GitHub Action for dependency auditing with depcheck and issue posting. And a separate Dependabot workflow for managing outdated dependencies.”
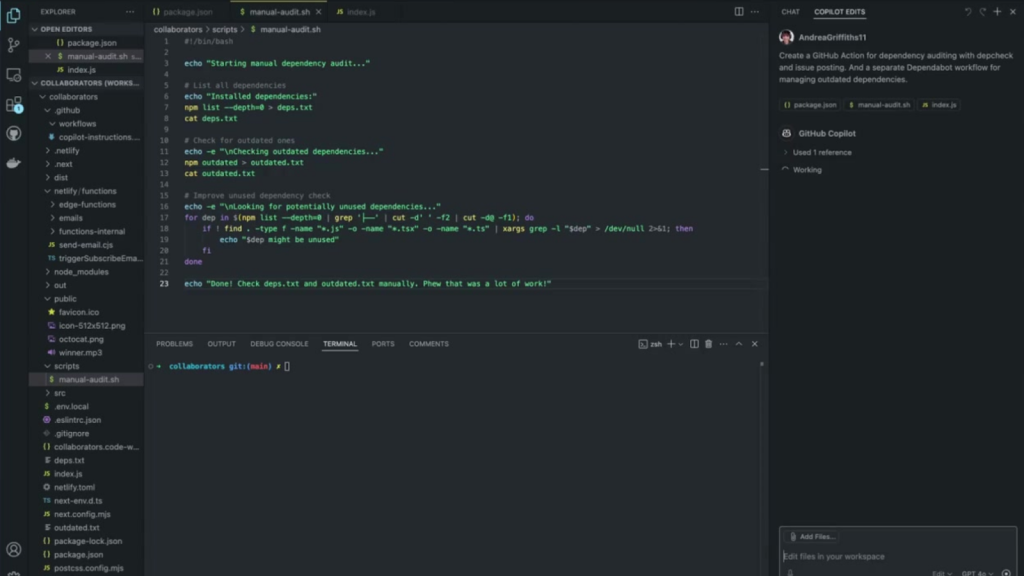
Remember our original bash script? With just a prompt, I shared the context (package.json and our manual script) and asked it to create an action that uses the dependency checker tool depcheck for more accurate detection of unused dependencies.
Step 2: GitHub Copilot writes the GitHub Action
To implement this GitHub Action, GitHub Copilot creates the new workflow file in .github/workflows/dependency-audit.yml
. Here’s the workflow file Copilot helped create:
name: Dependency Audit
on:
schedule:
- cron: '0 0 * * 1'
workflow_dispatch:
jobs:
audit:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Set up Node.js
uses: actions/setup-node@v4
with:
node-version: '18'
- name: Install dependencies
run: npm ci
- name: Install depcheck
run: npm install -g depcheck
- name: Run depcheck for unused dependencies
run: depcheck --json > unused-deps.json
- name: Run npm audit
run: npm audit --json > security-audit.json
- name: Generate report
run: |
echo "# Dependency Audit Report $(date)" > report.md
echo "## Unused Dependencies" >> report.md
cat unused-deps.json | jq -r '.dependencies[]' >> report.md
echo "## Security Issues" >> report.md
cat security-audit.json | jq '.metadata.vulnerabilities' >> report.md
- name: Create issue if problems found
uses: peter-evans/create-issue-from-file@v4
if: ${{ success() }}
with:
title: Weekly Dependency Audit
content-filepath: ./report.md
labels: maintenance, dependencies
Step 3: Enable Dependabot
While our custom action focuses on finding unused dependencies, we can use Dependabot to automatically create pull requests for outdated packages. Dependabot can be configured either via a simple YAML file or automatically by turning it on from your repository settings. Here’s the YAML file that Copilot created:
version: 2
updates:
- package-ecosystem: "npm"
directory: "/"
schedule:
interval: "weekly"
open-pull-requests-limit: 10
The result: a fully automated dependency audit
With that, our dependency management is now fully automated. Let’s recap how it works:
- Our custom action uses depcheck to accurately identify unused dependencies.
- Dependabot creates pull requests for outdated packages, complete with changelogs and risk assessments.
- Security vulnerabilities are detected and reported weekly.
- Everything is documented in GitHub Issues for team visibility.
This approach not only saves time but also significantly reduces the security risks and performance issues that stem from poorly managed dependencies.
By combining the AI capabilities of GitHub Copilot with GitHub Actions and Dependabot, we’ve turned a tedious manual task into an automated, integrated solution that keeps our codebase lean and secure. And I’ll take those time savings to do something more interesting, like unraveling the mystery of those weird goats in the TV show Severance. Or who knows, maybe I’ll finally figure out what macrodata refinement even means in that show (does anyone have any idea yet? Season two is killing me).
Try GitHub Copilot free and activate Dependabot
Learn more about GitHub Copilot for Business or start your free trial of GitHub Enterprise today.
Written by
Andrea is a Senior Developer Advocate at GitHub with over a decade of experience in developer tools. She combines technical depth with a mission to make advanced technologies more accessible. After transitioning from Army service and construction management to software development, she brings a unique perspective to bridging complex engineering concepts with practical implementation. She lives in Florida with her Welsh partner, two sons, and two dogs, where she continues to drive innovation and support open source through GitHub's global initiatives. Find her online @alacolombiadev.