Keep reading for the top 12 Git commands you’ll use every day.
- Git config
After you install Git on your machine, the first thing you should do is configure Git so that it understands who you are. The git config
command allows you to set Git configuration values that can help you create a customized Git workflow. To set your email and username, type git config --global user.name "username"
and git config --global user.email "youremail@email.com"
and these two settings allow Git to associate your work with your username and email, so you can get credit for the work that you do.

-
Git init
Like we mentioned before, the git init
command is used to initialize a new Git repository. Think of it as turning on a Git switch.
Let’s say, for example, you create a new folder with the terminal command mkdir project1
. You can go into project1
by running cd project1
. In its current state, project1
is not a Git repository.
If you want this folder to be a Git repository so that you can track all changes you make, type git i
with the alias “I” created earlier for the git init
command. Alternatively, you can just type git init
if you don’t have an alias set.

When you run the git init
command in an ordinary folder, it allows you to transform that folder into a trackable git repository where you can use Git commands.
-
Git status
If you want to see what you’ve done so far in your new Git repository, you can type git status
, which allows you to see what files have been added, deleted, or modified in your working directory. In this case, since you haven’t added any files yet, this should return “nothing to commit.”
Let’s create a new file by running touch hello.md
then run git status
again. Now you should see that you have an untracked file “hello.md”.

An untracked file is one that has not been added to the staging area. To add files to the staging area, you can use the git add
command.
-
Git add
There are quite a few ways to use this command. For example, you can use git add .
to add all files to the staging area from the working directory or use git add filename
to add a specific file to the staging area.
Let’s create a new file called learning.py
and another file called waiting.py
. Now, let’s run git status
so you can see your changes, then run git add learning.py
to add this file to the staging area. If you run Git status again, this will show you that learning.py
is in the staging area and waiting.py
is untracked in the working directory.

When files are added to the staging area, that means they are in safe keeping before you commit them. Think of using the Git add command as telling Git, “Please keep track of this file in its current state.”
If you make additional changes to the tracked files, you’ll need to use the git add
command again for Git to keep track of them. Add some code to the learning.py
file so you can see what we mean.
Currently, learning.py
and hello.md
files are being tracked in the staging area but waiting.py
is currently untracked. If you add the code print ("I'm learning git")
to learning.py
, then run git status
in the terminal, you’ll see that the learning.py
file has been modified and has untracked changes. To keep track of these newer changes, run git add learning.py
.

In the real world, you would typically complete the work you’re doing in the learning.py
file and then add those changes to the staging area. Once you’re satisfied with your work, you can then commit your changes.
-
Git commit
To “commit” a change is to store a version of your project in the Git history.
Currently, learning.py
, and hello.md
are tracked, while waiting.py
is untracked. Let’s run git commit -m "initial commit"
to see what happens.
If you run git status
, you’ll see that you have “2 files changed, 1 insertion in git” because you just added two new files, and one of the files has one line of code. You will also see that you have an untracked file—waiting.py
—because that file was never added to the staging area.
If you add new changes to the learning.py
file, you can add both files to the staging area, and along with any other changes you make, by running git add. git commit -m "add waiting file and new function"
command.

That’s how you use the git add
and git commit
commands together! Well done tracking changes and storing your new work.
-
Git clone
What if you were given a link to a folder from a remote location that you needed to get on your laptop? How would you do that? You’d need to make a copy of the remote repository to your local machine. In the world of Git, this is referred to as “cloning.” The command for cloning is, you guessed it, git clone
.
When you work on a team that uses Git for collaboration, you may be asked to use Git to make a copy of a project folder to make changes.
Say, for example, you have a remote repository on GitHub. To make a copy or clone this repository to your machine, click on the green “Code” button, then select the option that says HTTPS. Click on the copy icon to copy the link to the repository, and then open up your terminal and type git clone <url link>
, paste the URL link in your terminal, and hit the enter/return key.

Once you see “done,” you just successfully cloned a repository to your machine. Now, you have your own local copy of that remote repository that you can work in and edit.
-
Git checkout
When working with a Git repository with your team, it’s important to add your changes to a new branch—a branch in a Git repository is like creating a copy of a document so you don’t mess up the original. Branches allow you to work more collaboratively and effectively with your teammates in the same project.
So, let’s create a new branch in the repository that you just cloned.

Type git checkout -b update-name
to create a new branch called update-name
that you can make your changes on. This command will allow you to create a new branch and switch to the branch at the same time.
-
Git branch
To see a list of the branches you have, you can type git branch
and it will show that you have three branches—the main
branch, a branch named init, and the newly created update-name
branch. Hit the q key on your keyboard to exit.
-
Git switch
Now, what if you wanted to go back to the main branch? You can use the git switch
command. In your terminal, type git switch main
to return to the main branch. Great, now you’re back on the main branch.
Let’s return to the branch you created and add a few changes to a file.
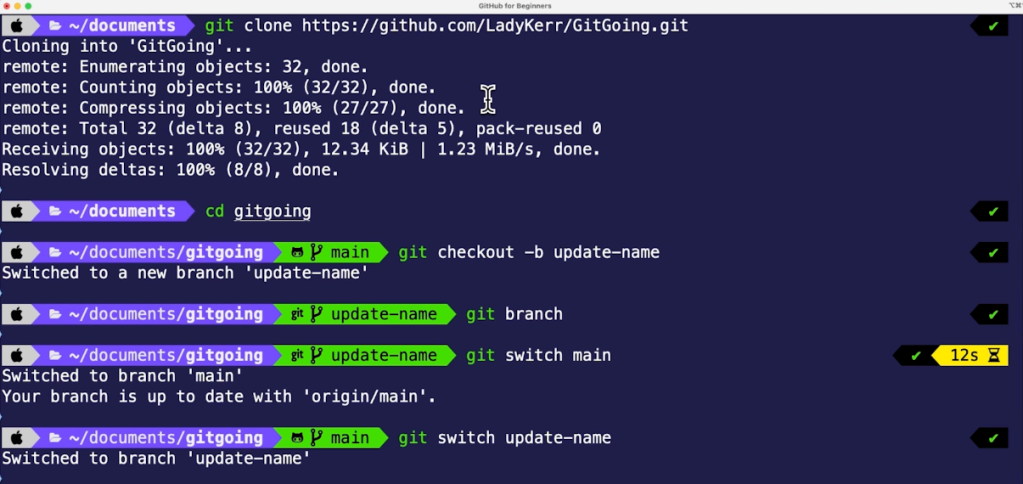
Type git switch update-name
and then open the project in your code editor. Navigate to the index.html
file, right click on the file, and select open preview
. This will open up a side browser where you can see the changes live in your code.
Let’s say you were tasked with changing the title of this app to “Git Going.” Let’s update the name in the HTML file and run git status
to see that the file has been modified.
Practicing what you learned earlier, add these changes to the staging area, then commit the changes to your local repository—git add . git commit -m "update app name" git status
. Now, get your local changes into the remote repository that you cloned earlier to update the app.
-
Git push
To get the changes you just made into the remote repository, you need to push your changes to the remote repository.
The command git push
tells Git, “Thanks for tracking these file changes. Now I want to upload these changes into the main project file.”
When you push your changes, you are essentially updating the remote repository with commits that have been made in your local repository. To do this, use the command git push <remote> <branch>
where “remote” refers to the remote repository, and “branch” refers to the branch you’re working on.
In your case, you can type git push origin update-name
where “origin” refers to the “remote repository” and “update-name” refers to the branch you want to upload.
If you go back to the repository on GitHub, you will see that there’s a new branch available. It’s been “pushed” up to the repository, which is why we call it Git push!

You can now open up a pull request on GitHub to merge your changes into the main branch. A pull request is a proposal to merge a set of changes from one branch into another. In your case, you want to merge the updated name into the main branch.
-
Git pull
git pull
is an important command to run to keep your local repository up to date with the remote repository.
Now that you’ve updated the main branch in the remote repository with your changes, go back to your local repository.
In the terminal, type git switch main
, then open up the file in your editor. Navigate to the index.html
file and check the name of the app. You’ll see that the app name is still “Todo” in the file even though you just updated the main branch with the new name. So, what happened? If you guessed that you updated the remote repository’s main branch and not your local copy, then you’re right.
Remember that a local repository is a copy/clone of your remote repository and each branch has its own history. Since you made the change on the local branch update-name
and pushed to the remote repository, you need to do the same thing locally and merge your changes into your local copy of the main
branch.
You can update your local branches with their remote counterparts with the command git pull
. This command will fetch all changes that were made and merge them into your local branch.

In the terminal, on the main branch, run git pull
. This will update your branch and return the changes that were made. You’ll then see that one file was changed with one insertion and one deletion.
So, you made a bunch of changes. How do you keep track of everything you’ve done so far?
You’re now fully equipped to work with git as a professional. Practicing these commands will make you a more effective Git user. And this leads us into what we call the GitHub flow.
You cloned the repository, created a new branch, made changes on that branch, pushed to GitHub, and opened up a pull request. Typically at this stage, you would request feedback from your teammates about the work that you did and then address comments. Then you’d merge the pull request into the main branch and delete your branch.
Let’s do the final stage of the GitHub flow and delete your branch. The command to delete a branch is git branch -d update-name
and this will delete the branch locally. To delete a branch remotely, you can run the next command git push —delete origin update-name
and this will delete the branch in the remote repository.
And that’s the GitHub flow.
You just learned 10 essential Git commands that you’ll be using every day on your journey as a developer. Keep practicing your Git skills by going through the GitHub flow and practicing the commands you just learned. If you have any questions or feedback, pop it in the GitHub Community thread and we’ll be sure to respond!